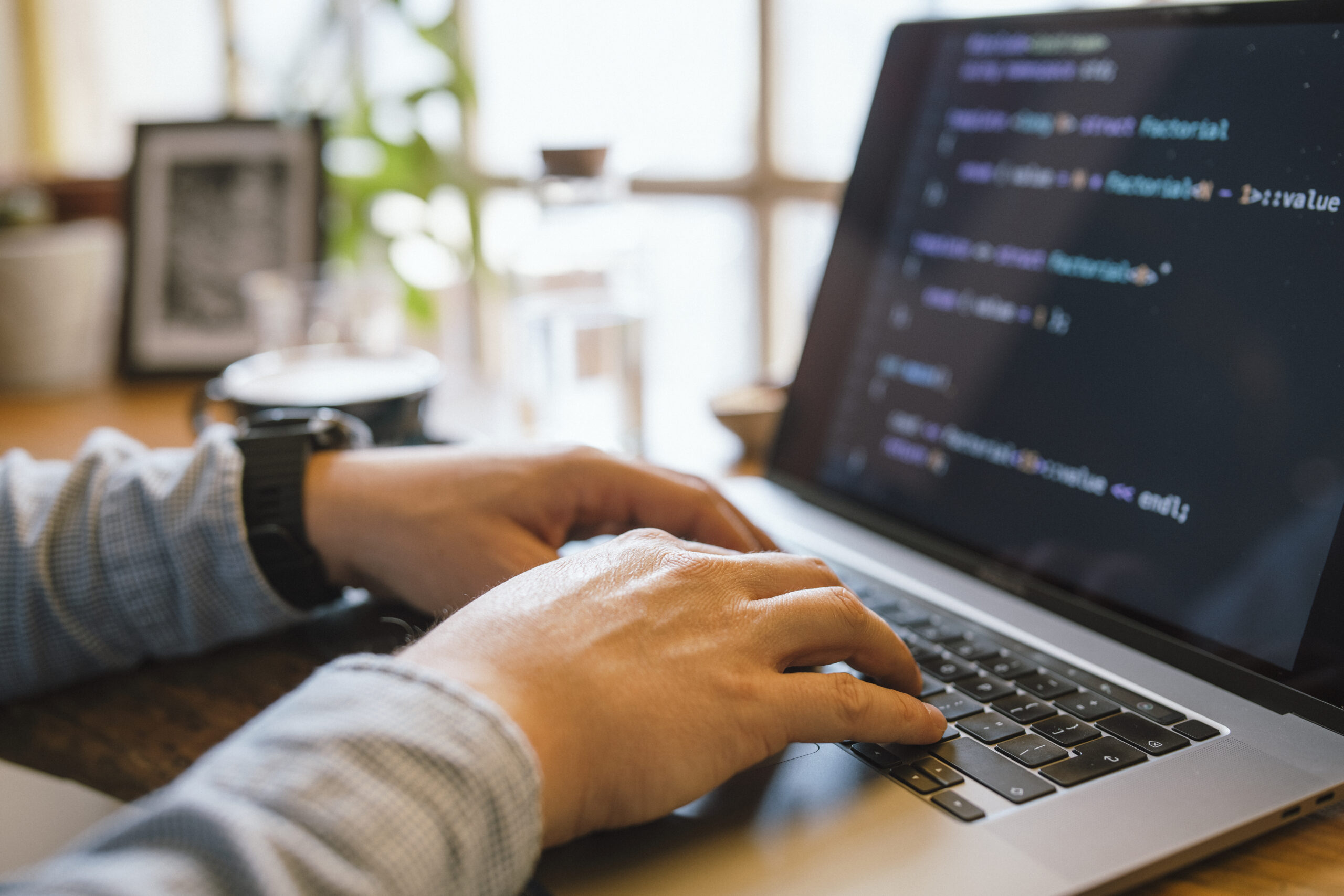
Debugging is Probably the most necessary — yet frequently disregarded — techniques in a very developer’s toolkit. It's actually not almost correcting damaged code; it’s about comprehending how and why items go Improper, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a rookie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably enhance your productivity. Here are quite a few procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest methods developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single A part of advancement, realizing how you can connect with it proficiently for the duration of execution is equally crucial. Modern enhancement environments arrive equipped with impressive debugging abilities — but quite a few developers only scratch the surface of what these instruments can do.
Consider, for example, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources let you set breakpoints, inspect the worth of variables at runtime, move by code line by line, and also modify code on the fly. When made use of the right way, they Allow you to observe exactly how your code behaves during execution, which happens to be priceless for tracking down elusive bugs.
Browser developer instruments, which include Chrome DevTools, are indispensable for front-close developers. They permit you to inspect the DOM, monitor network requests, perspective genuine-time functionality metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can switch irritating UI difficulties into workable responsibilities.
For backend or procedure-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control about operating procedures and memory administration. Learning these applications may have a steeper learning curve but pays off when debugging performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become snug with version Manage programs like Git to be aware of code history, locate the exact instant bugs were introduced, and isolate problematic adjustments.
In the long run, mastering your resources suggests heading further than default settings and shortcuts — it’s about establishing an personal understanding of your progress environment in order that when difficulties crop up, you’re not dropped in the dark. The higher you recognize your tools, the more time you are able to invest solving the actual problem rather than fumbling by means of the process.
Reproduce the issue
Just about the most vital — and often ignored — steps in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, builders want to make a steady atmosphere or scenario where the bug reliably seems. With no reproducibility, fixing a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps resulted in the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the less complicated it gets to be to isolate the precise circumstances below which the bug takes place.
After you’ve gathered ample details, try to recreate the situation in your local ecosystem. This might necessarily mean inputting precisely the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If The problem seems intermittently, think about composing automated assessments that replicate the edge circumstances or point out transitions involved. These assessments don't just enable expose the issue and also reduce regressions Sooner or later.
Occasionally, The problem can be environment-unique — it might take place only on selected operating techniques, browsers, or underneath individual configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t just a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you are previously midway to correcting it. Which has a reproducible state of affairs, You should use your debugging applications more efficiently, take a look at opportunity fixes properly, and connect extra Evidently with all your workforce or consumers. It turns an abstract complaint into a concrete obstacle — Which’s where by builders prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. Rather then looking at them as annoying interruptions, builders must find out to treat mistake messages as immediate communications through the program. They frequently show you just what exactly took place, in which it happened, and in some cases even why it transpired — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of builders, especially when less than time strain, glance at the 1st line and quickly begin earning assumptions. But further inside the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read and fully grasp them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic error? Will it point to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and issue you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable designs, and Discovering to recognize these can greatly quicken your debugging course of action.
Some errors are obscure or generic, As well as in These scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized problems and provide hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and become a far more efficient and assured developer.
Use Logging Correctly
Logging is Probably the most impressive resources within a developer’s debugging toolkit. When utilized properly, it offers authentic-time insights into how an software behaves, helping you comprehend what’s happening under the hood without needing to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges include DEBUG, Data, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Data for standard functions (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual issues, and FATAL if the technique can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and slow down your system. Deal with essential occasions, point out alterations, input/output values, and important selection factors in your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specially valuable in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Just like a Detective
Debugging is not merely a technical process—it is a form of investigation. To efficiently establish and fix bugs, developers need to approach the process just like a detective fixing a secret. This mindset assists break down advanced challenges into manageable areas and abide by clues logically to uncover the root lead to.
Get started by gathering evidence. Consider the indicators of the condition: mistake messages, incorrect output, or effectiveness challenges. Just like a detective surveys a crime scene, gather just as much applicable info as it is possible to with out jumping to conclusions. Use logs, test situations, and person reports to piece with each other a clear image of what’s taking place.
Following, sort hypotheses. Talk to oneself: What may be resulting in this actions? Have any variations a short while ago been produced towards the codebase? Has this problem occurred before beneath related conditions? The objective would be to slender down options and discover possible culprits.
Then, test your theories systematically. Seek to recreate the issue inside of a managed surroundings. If you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code inquiries and Allow the outcomes lead you nearer to the reality.
Spend shut focus to little aspects. Bugs typically conceal in the minimum expected destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and patient, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might cover the actual difficulty, just for it to resurface later.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people have an understanding of your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Publish Assessments
Crafting tests is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Tests not just aid capture bugs early and also function a security Web that offers you assurance when making modifications in your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where read more and when a problem occurs.
Start with device checks, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as predicted. Every time a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following Formerly being preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist make sure several areas of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you may need to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust initial step. When the test fails persistently, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Taking breaks helps you reset your mind, decrease disappointment, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code which you wrote just hours earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the root of a dilemma when they've taken the perfect time to disconnect, permitting their subconscious perform inside the background.
Breaks also assistance protect against burnout, Specifically throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to set a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it basically results in speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It provides your Mind space to breathe, enhances your standpoint, and assists you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of critical thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better methods like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or comprehending and assist you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring challenges or prevalent issues—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, assisting others avoid the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the finest developers are certainly not those who write best code, but those that repeatedly learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, more capable developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become far better at Anything you do.